Leo's World Through Articles
Stay up-to-date on my coding journey and find out what's going on in my world.
- ✔️New Technologies
- ✔️Project Building
- ✔️Personal Development
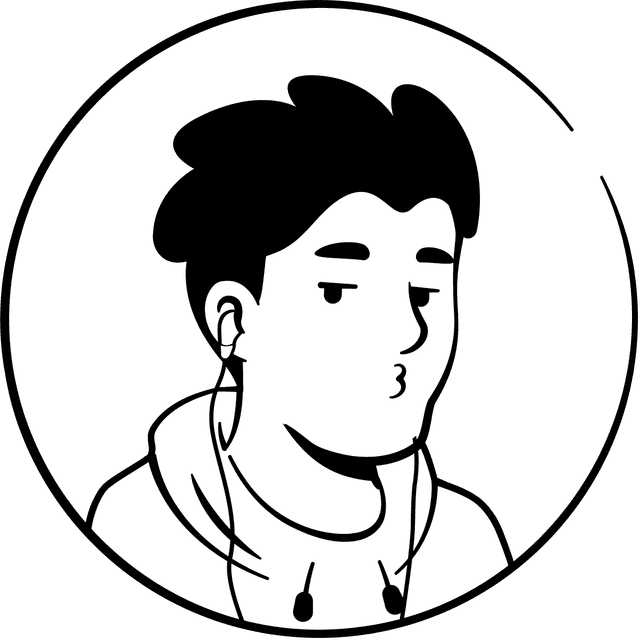
Trending
HTTP Errors: 404
An introduction to the Web's most popular error Background: There are five types of HTTP status codes that report on communications between the client and server. The 400-level codes denote a client-side error, meaning an error that originated from an action taken by the user of a web app. Sometimes you’ll see a 404 error if you incorrectly type the URL to a web page you want to visit, and instead of being taken there, you’re rerouted to a page that reads something like, “404 Error - File Not Found.” The 404 error is telling you that the request you sent did in fact make it to the server, but the server could not find the specific file you were requesting. Other actions that will set off a 404 error: Clicking a link to a file that has been deleted or renamed Using Your Browser’s Developer Tools to Check Status Codes: Many modern browsers have a “developer tools” mode that allows you to access loads of meta-data about web pages. Here’s how to use Google Chrome’s Developer Tools to check the status codes of files on the web. (Other browsers’ developer tools have this feature too, but you’ll have to google those to learn more.) Instructions: If on a Mac, go to a web page of your choosing and press down the control button while you click anywhere on the document. If you’re on a PC, just right-click anywhere in the document. A menu will pop up next to where you clicked. Click the “Inspect Element” option. The developer tools panel will show up in the bottom third of your screen. Don’t be afraid! It will go away if you click the small “x” at the top far-right of the panel. To check status codes of the files listed on the web page, click on the “Network” tab (the second tab from the right, directly after “Elements”). On the bottom half of the panel for this tab, you’ll see a table listing information about all the files associated with that web page. The “Status” column will show you the HTTP status of each file. Do some exploring! Click buttons and links on the web page you’ve visited, and see what changes in the “Network” tab. If you see a status code written in red, it means there was an error. Maybe it’s a 404. Check it out!
Getting User Input in Node.js
Learn how to handle user input synchronously in Node.js. Synchronous Input Node.js allows you to run JavaScript code outside of a browser window, offering powerful tools to interact with a computer filesystem, run web servers, and create terminal applications. Node handles these tasks by running asynchronously, which means that reading user input from a terminal isn’t as simple as calling a getInput() function. In this article, we’ll show you an easy way around that (and some tips and tricks for handling user input) by using a helpful Node module. Working with Input Node.js provides a few ways to handle interactions, including the built-in process object and readline module. While these are powerful tools, they rely on callback functions and can be confusing to work with at first. const readline = require('readline').createInterface({ input: process.stdin, output: process.stdout }); readline.question('Who are you?', name => { console.log(`Hey there ${name}!`); readline.close(); }); Running this code in Node, you’d see: $ node read.js Who are you? > Then you could enter your input based on the prompt and see the response: $ node read.js Who are you? > Codecademy Hey there Codecademy! $ This works as intended, but it’s a lot of boilerplate, and you need to call readline.question() and create a callback function every time you want to use it for input. There’s a simpler way, whether you’re just getting started out with JavaScript development or just want to get an interactive script running as quickly as possible. Using prompt-sync The prompt-sync Node module provides an easy-to-use alternative to this callback-based syntax. Make sure you have Node and NPM installed Run npm install prompt-sync in the terminal const prompt = require('prompt-sync')(); Notice the extra () after require(). The prompt-sync module is a function that creates prompting functions, so you need to call prompt-sync in order to get your actual prompting function. Once you’ve loaded the prompt-sync module and called it, using it to retrieve user input is relatively straightforward: const prompt = require('prompt-sync')(); const name = prompt('What is your name?'); console.log(`Hey there ${name}`); The prompt() function returns the user feedback, so simply store that return value to a variable to use it later. In the example above, the name variable stores the value, and it is then repeated to the user on the next line. Letting Users Exit By default, most terminal programs will exit with Ctrl + C (This sends a SIGINT, or “signal interrupt” message indicating that a user wants to exit a program). With prompt-sync, in order to make sure that your users can exit at will, add a configuration object with sigint: true when invoking the prompt-sync function: const prompt = require('prompt-sync')({sigint: true}); Working with Numbers All user input will be read as a String, so in order to treat user input as numbers, you’ll need to convert the input: const num = prompt('Enter a number: '); console.log('Your number + 4 ='); console.log(Number(num) + 4); Making a basic app The code below creates a small number guessing application. const prompt = require('prompt-sync')({sigint: true}); // Random number from 1 - 10 const numberToGuess = Math.floor(Math.random() * 10) + 1; // This variable is used to determine if the app should continue prompting the user for input let foundCorrectNumber = false; while (!foundCorrectNumber) { // Get user input let guess = prompt('Guess a number from 1 to 10: '); // Convert the string input to a number guess = Number(guess); // Compare the guess to the secret answer and let the user know. if (guess === numberToGuess) { console.log('Congrats, you got it!'); foundCorrectNumber = true; } else { console.log('Sorry, guess again!'); } }
Deploying to Github Pages
GitHub is a great tool to store projects and to collaborate with others, but its usefulness does not stop there. We’ll use a service called GitHub Pages to share our web page creations on the World Wide Web. What is GitHub Pages? There are many different ways to deploy a website to the public Internet. We’ll be using GitHub’s free service called GitHub Pages. Why GitHub Pages? GitHub Pages offers a lot of features and flexibility, all for free. Some of the benefits include: Easy setup Seamless collaboration using Git and GitHub Free hosting with >95% uptime Live updating with normal GitHub workflow What is Deploying? Deploying is like publishing. When authors are ready for their work to be seen by the world, they publish it. When web developers are ready to share their projects, they deploy to the World Wide Web. Deployment is when a project is packaged and shared on the Internet. Unlike publishing, however, deployment may occur many, many times over the course of a software project. Deployment on GitHub Pages Deploying to GitHub Pages is automatic. Once it’s set up, deploying happens whenever you push your local changes to your remote, GitHub-hosted repository. Head to GitHub Pages’ setup instructions and follow the steps exactly to get your main GitHub Pages page setup. When you first navigate to your newly deployed site it is possible that you will receive a 404 error. If this happens, and you are confident that you have followed all the steps as written, check back in 30 minutes to see if the deploy has successfully gone through. Viewing Your Live Web Page That’s it! Your website is deployed to the Internet! You and anyone with whom you share this link can view your project by navigating in your browser to the URL http://<your-github-username>.github.io. Adding GitHub Pages Projects You can set up your GitHub Pages to deploy every one of your repositories in addition to <username>.github.io. This will allow you to ensure all of your sites are deployed automatically whenever you push to GitHub. In GitHub, navigate to your <username>.github.io repository and click Settings. githubSettings Within Settings, navigate to the Source section within the Github Pages section. From the dropdown menu, select master branch and then click Save. githubPagesSection Now, all of your repositories can be found at http://<username>.github.io/<repository-name>. Try creating a new repo with an HTML project inside it (perhaps push an old project to GitHub) and then navigate to the deployed page. Deploying New Changes Now that your GitHub Pages site is set up, deploying new changes is easy. Every time you make a change to your site, use the normal GitHub flow. That is, use git commit and git push to send your changes to GitHub. After this, the GitHub site should update within a few seconds. Just refresh the page in your browser, and you’re good to go! Congratulations on your first live web page!
Stay in the Know
Stay up-to-date with the latest tech news and announcements by reading our curated articles, ranging from bite-sized pieces to in-depth features. Perfect for busy users who want to stay informed on the go and for those who prefer to dive deep into a topic.